Bjarne Stroustrup
Before the initial standardization in 1998, C++ was developed by Danish computer scientist Bjarne Stroustrup at Bell Labs since 1979 as an extension of the C language; he wanted an efficient and flexible language similar to C that also provided high-level features for program organization.
C++ Tutorial
C++ is a popular programming language.
C++ is used to create computer programs.
Examples in Each Chapter
#include
ANSWERS
Hello World!
We recommend reading this tutorial, in the sequence listed in the left menu.
हम इस ट्यूटोरियल को बाएं मेनू में सूचीबद्ध अनुक्रम में पढ़ने की सलाह देते हैं
C++ is an object oriented language and some concepts may be new. Take breaks when needed, and go over the examples as many times as needed.
C ++ एक ऑब्जेक्ट ओरिएंटेड भाषा है और कुछ अवधारणाएँ नई हो सकती हैं। जरूरत पड़ने पर ब्रेक लेते हैं, और आवश्यकतानुसार कई बार उदाहरणों पर चलते हैं
C++ Introduction
What is C++?
C++ is a cross-platform language that can be used to create high-performance applications.
C ++ एक क्रॉस-प्लेटफ़ॉर्म भाषा है जिसका उपयोग उच्च-प्रदर्शन अनुप्रयोगों को बनाने के लिए किया जा सकता है।
C++ was developed by Bjarne Stroustrup, as an extension to the C language.
C ++ को Bjarne Stroustrup द्वारा C भाषा के विस्तार के रूप में विकसित किया गया था।
C++ gives programmers a high level of control over system resources and memory.
C ++ प्रोग्रामर्स को सिस्टम संसाधनों और मेमोरी पर उच्च स्तर का नियंत्रण देता है।
The language was updated 3 major times in 2011, 2014, and 2017 to C++11, C++14, and C++17.
Why Use C++
C++ is one of the world's most popular programming languages.
C++ can be found in today's operating systems, Graphical User Interfaces, and embedded systems.
C++ is an object-oriented programming language which gives a clear structure to programs and allows code to be reused, lowering development costs.
C++ is portable and can be used to develop applications that can be adapted to multiple platforms. C++ is fun and easy to learn!
As C++ is close to C# and Java, it makes it easy for programmers to switch to C++ or vice versa
C ++ दुनिया की सबसे लोकप्रिय प्रोग्रामिंग भाषाओं में से एक है।
C ++ आज के ऑपरेटिंग सिस्टम, ग्राफिकल यूजर इंटरफेस और एम्बेडेड सिस्टम में पाया जा सकता है।
C ++ एक ऑब्जेक्ट-ओरिएंटेड प्रोग्रामिंग लैंग्वेज है जो कार्यक्रमों को एक स्पष्ट संरचना देती है और कोड को पुन: उपयोग करने की अनुमति देती है, जिससे विकास लागत कम होती है।
C ++ पोर्टेबल है और इसका उपयोग उन अनुप्रयोगों को विकसित करने के लिए किया जा सकता है जिन्हें कई प्लेटफार्मों पर अनुकूलित किया जा सकता है।
C ++ मजेदार और सीखने में आसान है!
चूंकि C ++ C # और Java के करीब है, इसलिए प्रोग्रामर के लिए C ++ या इसके विपरीत स्विच करना आसान हो जाता है
C++ Getting Started
C++ Get Started
To start using C++, you need two things:
A text editor, like Notepad, to write C++ code
A compiler, like GCC, to translate the C++ code into a language that the computer will understand
There are many text editors and compilers to choose from. In this tutorial, we will use an IDE (see below
C ++ शुरू हो जाओ
C ++ का उपयोग शुरू करने के लिए, आपको दो चीजों की आवश्यकता है:
एक पाठ संपादक, नोटपैड की तरह, सी ++ कोड को लिखने के लिए
एक संकलक, जीसीसी की तरह, सी ++ कोड को एक ऐसी भाषा में अनुवाद करने के लिए जिसे कंप्यूटर समझ जाएगा
चुनने के लिए कई पाठ संपादक और संकलनकर्ता हैं। इस ट्यूटोरियल में, हम एक IDE (नीचे देखें) का उपयोग करेंगे
C++ Install IDE
An IDE (Integrated Development Environment) is used to edit AND compile the code.
Popular IDE's include Code::Blocks, Eclipse, and Visual Studio. These are all free, and they can be used to both edit and debug C++ code.
Note: Web-based IDE's can work as well, but functionality is limited.
We will use Code::Blocks in our tutorial, which we believe is a good place to start.
कोड को संपादित करने और संकलित करने के लिए एक आईडीई (एकीकृत विकास पर्यावरण) का उपयोग किया जाता है।
लोकप्रिय आईडीई के कोड :: ब्लॉक, ग्रहण और दृश्य स्टूडियो शामिल हैं। ये सभी मुफ़्त हैं, और इन्हें C ++ कोड को संपादित और डीबग करने के लिए उपयोग किया जा सकता है।
नोट: वेब-आधारित IDE के रूप में अच्छी तरह से काम कर सकते हैं, लेकिन कार्यक्षमता सीमित है।
हम अपने ट्यूटोरियल में कोड :: ब्लॉक का उपयोग करेंगे, जो हमें लगता है कि शुरू करने के लिए एक अच्छी जगह है।
C++ Quickstart
Let's create our first C++ file.
Open Codeblocks and go to File > New > Empty File.
Write the following C++ code and save the file as myfirstprogram.cpp (File > Save File as):
myfirstprogram.cpp
#include
चलिए हमारी पहली C ++ फाइल बनाते हैं। कोडब्लॉक खोलें और फाइल> न्यू> खाली फाइल पर जाएं। निम्नलिखित C ++ कोड लिखें और फ़ाइल को myfirstprogram.cpp के रूप में सहेजें (फ़ाइल> फ़ाइल को इस रूप में सहेजें):
Don't worry if you don't understand the code above - we will discuss it in detail in later chapters. For now, focus on how to run the code.
In Codeblocks, it should look like this
यदि आप ऊपर दिए गए कोड को नहीं समझते हैं तो चिंता न करें - हम बाद के अध्यायों में इसके बारे में विस्तार से चर्चा करेंगे। अभी के लिए, कोड को चलाने के तरीके पर ध्यान दें।
कोडब्लॉक में, यह इस तरह दिखना चाहिए
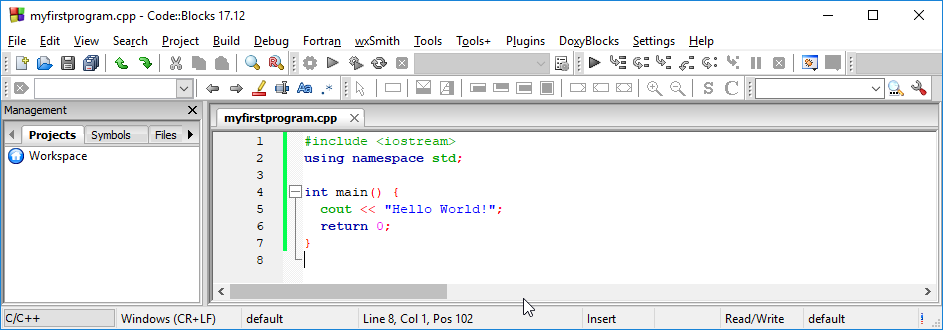
Then, go to Build > Build and Run to run (execute) the program. The result will look something to this:
Hello World!
Process returned 0 (0x0) execution time : 0.011 s
Press any key to continue.
myfirstprogram.cpp Code:
#include
using namespace std;
int main() {
cout << "Hello World!";
return 0;
}
Result:
Hello World!
1 Comments